How to use NLog LayoutRenderer in ASP.NET Core
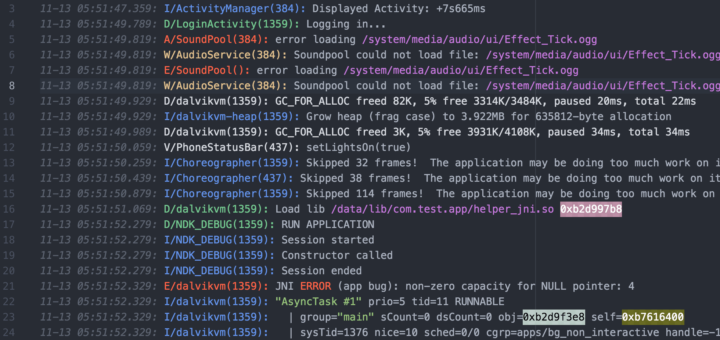
This time I like to share the way to use NLog LayoutRenderer in ASP.NET Core, According to NLog website:
NLog is a flexible and free logging platform for various .NET platforms, including .NET standard. NLog makes it easy to write to several targets. (database, file, console) and change the logging configuration on-the-fly.
If you love NLog and the way it facilitates logging in ASP.NET, then you might wonder how easy it is to use a custom LayoutRenderer.
First, let’s take a look at how can we setup NLog in an ASP.NET Core 2.0 application.
-
- Create a new ASP.NET Core application and select everything as default
- Install the following NLog libraries from nuget package manager.
- NLog.Web.AspNetCore 4.5+
- Create a new config file for nlog, this will help to isolate nlog configurations, let’s name it nlog.config. Paste the following code. The configuration file is very straightforward as before, almost nothing changed.
<?xml version="1.0" encoding="utf-8" ?> <nlog xmlns="http://www.nlog-project.org/schemas/NLog.xsd" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" autoReload="true" internalLogLevel="info" internalLogFile="c:\temp\internal-nlog.txt"> <!-- enable asp.net core layout renderers --> <extensions> <add assembly="NLog.Web.AspNetCore"/> </extensions> <!-- the targets to write to --> <targets> <!-- write logs to file --> <target xsi:type="File" name="allfile" fileName="c:\temp\nlog-all-${shortdate}.log" layout="${longdate}|${event-properties:item=EventId_Id}|${uppercase:${level}}|${logger}|${message} ${exception:format=tostring} ${session-id} " /> <!-- rules to map from logger name to target --> <rules> <!--All logs, including from Microsoft--> <logger name="*" minlevel="Trace" writeTo="allfile" /> <!--Skip non-critical Microsoft logs and so log only own logs--> <logger name="Microsoft.*" maxLevel="Info" final="true" /> <!-- BlackHole without writeTo --> <logger name="*" minlevel="Trace" writeTo="ownFile-web" /> </rules> </nlog>
4. In your program.cs add UseNLog() to DI container, you need to import using Microsoft.Extensions.Logging and NLog.Web.
Imagine you want a new template for tracking a session-id in your application, so, to do that create a simple class and decorate that with [LayoutRenderer(“session-id”)]
[LayoutRenderer("error-data")] public class ErrorDataLayoutRenderer : AspNetLayoutRendererBase { protected override void DoAppend(StringBuilder builder, LogEventInfo logEvent) { var session = logEvent.Properties.ContainsKey("session-id") ? logEvent.Properties["session-id"] as string : null; if (!string.IsNullOrEmpty(session)) builder.Append(session); } }
NLog will pick your layout renderer definition automatically and you don’t need to do it manually. Next, get the logger in your class:
private readonly Logger _log = LogManager.GetLogger(typeof(HomeController).FullName);
Log what you need to log!
var info = new LogEventInfo() { Level = LogLevel.Info, Message = "THIS IS A TEST" }; info.Properties["session-id"] = "323I894j"; _log.Log(info);
Remeber you need to add ${session-id} into your config file.
That’s it, you used NLog LayoutRenderer in ASP.NET Core, let me know if you have any question. 🙂
Where did you exactly add ${session-id} ? I gone through your code, I couldn’t get it to work, it does not write my log info to the file
Thanks