How to test a secure API in Visual Studio
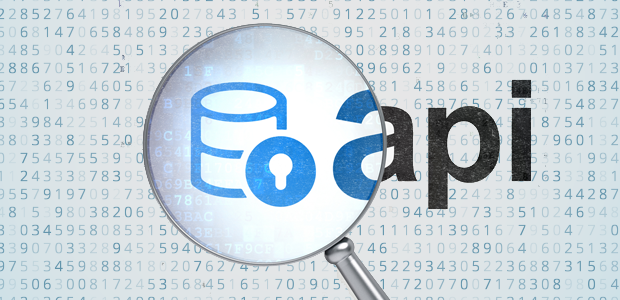
A typical way to test a secure API in Visual Studio is using bearer tokens, as JWT. In case you’re utilizing JWT, you may discover this site valuable to effortlessly look at JWT token substance. A typical situation when working with APIs security by bearer tokens is to need to accomplish something like this:
One way is to post the credentials to the authentication service to get a token. The other way is to pass a token to the server to get your request validated.
Usually, if you design your API properly, it should return Unauthorized(401) status code if the token is invalid
You could easily test this by using some tools like Postman and setting an invalid authorization header. First, pass your credential to get a token then on the second call just change your token slightly to see the 401 Unauthorized response.
It is crucial to load test your APIs to verify if your code is able to handle heaving loads of requests.
Remember calling an API to do authentication many times should not produce a new token each time, instead, your Auth service should have some logic in place to add expiry to your token whether it is a JWT token or a reference token.
In your test simply use the same token and load test your API, depending on token expiry you can renew the token when it is expired.
var client = new RestClient("http://yoursecureapi.com"); client.Authenticator = new HttpBasicAuthenticator(username, password); var request = new RestRequest("resource/{id}", Method.GET); // Add some header here maybe request.AddHeader("header", "value"); // run the request as many times IRestResponse response = await client.ExecuteAsync(request);
And that is how you can test a secure API in Visual Studio unit test.
This is especially very handy when you don’t have any external load testing component in place.
let me know about your ideas.